Complete DevOps CI/CD Pipeline with GitHub Actions, Docker & Kubernetes (Step-by-Step Guide)
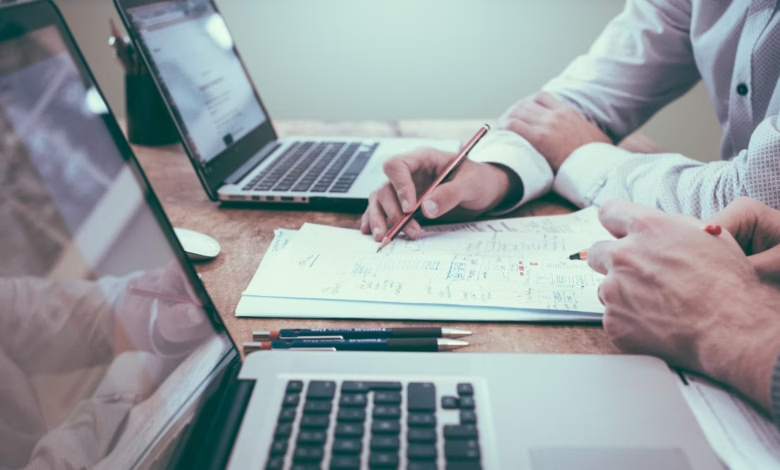
In today’s fast-paced software development environment, delivering high-quality software rapidly and reliably is critical. That’s where DevOps practices shine. This guide will walk you through the complete setup of a CI/CD pipeline using GitHub Actions, Docker, and Kubernetes, transforming your code from a simple push into a live deployment on a scalable Kubernetes cluster.
Whether you are a developer looking to level up your DevOps skills or a DevOps engineer building pipelines for teams, this tutorial will give you a production-grade example to work with.
Table of Contents
- What is CI/CD in DevOps?
- Tools Overview: GitHub Actions, Docker, Kubernetes
- Project Setup: Sample App Overview
- Step 1: Dockerizing Your Application
- Step 2: Writing a GitHub Actions Workflow
- Step 3: Setting Up a Kubernetes Cluster (Using Minikube or Cloud)
- Step 4: Deploying to Kubernetes
- Step 5: Automating the Entire Flow
- Securing the Pipeline (Secrets, Best Practices)
- Monitoring, Logging and Rollbacks
- Tips for Production-Grade Pipelines
- Final Thoughts
1. What is CI/CD in DevOps?
Continuous Integration (CI) is the process of automatically building and testing code every time a developer pushes to the repository. CI ensures that new changes integrate smoothly with the existing codebase, catching bugs early and increasing confidence in every push.
Continuous Deployment/Delivery (CD) takes it further by automatically deploying the changes to production or staging environments. This allows organizations to deliver software faster and more reliably.
Together, CI/CD forms the core of modern DevOps, reducing manual effort, human error, and increasing deployment velocity. The ultimate goal is to automate as much as possible, allowing developers to focus on building features instead of managing infrastructure.
2. Tools Overview
Let’s look at the tools we’ll be using to create this full pipeline.
✅ GitHub Actions
GitHub Actions is a powerful CI/CD tool integrated into GitHub. It lets you define custom workflows to build, test, and deploy your code based on GitHub events like push
, pull_request
, or even cron schedules.
Advantages:
- Native to GitHub
- Huge community and pre-built actions
- Easy to manage secrets and environments
- Free tier for public and private repos
✅ Docker
Docker allows us to package applications and their dependencies into containers. Containers are lightweight and ensure consistent environments across development, testing, and production.
Why use Docker?
- Eliminates “it works on my machine” issues
- Easy scaling and isolation
- Works perfectly with Kubernetes
✅ Kubernetes
Kubernetes (also known as K8s) is a container orchestration platform. It handles automatic deployments, scaling, load balancing, and rollbacks for containerized applications.
Why Kubernetes?
- Production-grade scaling
- Built-in support for health checks, secrets, config management
- Cloud-agnostic (works with AWS, GCP, Azure, or local setups)
3. Project Setup
We’ll use a sample web app (Node.js or .NET Core API) for demonstration purposes. The structure:
/app
|-- Dockerfile
|-- .github/workflows/deploy.yml
|-- k8s/
| |-- deployment.yaml
| |-- service.yaml
|-- src/
|-- app.js or Program.cs
To keep things clean, we’ll organize Kubernetes manifests in a k8s
folder and keep the CI/CD configuration in .github/workflows
.
Prerequisites:
- GitHub account
- Docker Hub account (or any container registry)
- Kubernetes cluster (Minikube, AKS, EKS, GKE, or k3s)
- Node.js or .NET Core installed
- kubectl configured locally
4. Step 1: Dockerizing Your App
Let’s create a simple Dockerfile that packages our app:
For Node.js
FROM node:18-alpine
WORKDIR /app
COPY . .
RUN npm install
CMD ["node", "src/app.js"]
EXPOSE 3000
For .NET Core
FROM mcr.microsoft.com/dotnet/aspnet:7.0 AS base
WORKDIR /app
COPY . .
ENTRYPOINT ["dotnet", "YourApp.dll"]
Build and Run Locally
docker build -t my-app .
docker run -p 3000:3000 my-app
You should now be able to test your app locally.
5. Step 2: Writing a GitHub Actions Workflow
Now we create a CI/CD workflow file.
File: .github/workflows/deploy.yml
name: CI/CD Pipeline
on:
push:
branches: [ main ]
jobs:
build-and-push:
runs-on: ubuntu-latest
steps:
- name: Checkout code
uses: actions/checkout@v3
- name: Log in to DockerHub
run: echo "${{ secrets.DOCKER_PASSWORD }}" | docker login -u ${{ secrets.DOCKER_USERNAME }} --password-stdin
- name: Build and push image
run: |
docker build -t mydockeruser/my-app:latest .
docker push mydockeruser/my-app:latest
You’ll need to store your DockerHub credentials in GitHub Secrets for security.
6. Step 3: Setting Up Kubernetes
For local development, we recommend Minikube:
minikube start
kubectl create deployment my-app --image=mydockeruser/my-app:latest
kubectl expose deployment my-app --type=NodePort --port=3000
minikube service my-app
For cloud:
- AWS EKS (with eksctl)
- Azure AKS (with az CLI)
- GCP GKE (with gcloud CLI)
Make sure kubectl
is configured and you have permissions to deploy.
7. Step 4: Kubernetes Manifests
Create deployment and service YAMLs in the k8s/
folder:
deployment.yaml
apiVersion: apps/v1
kind: Deployment
metadata:
name: my-app
spec:
replicas: 2
selector:
matchLabels:
app: my-app
template:
metadata:
labels:
app: my-app
spec:
containers:
- name: my-app
image: mydockeruser/my-app:latest
ports:
- containerPort: 3000
service.yaml
apiVersion: v1
kind: Service
metadata:
name: my-app-service
spec:
selector:
app: my-app
type: NodePort
ports:
- port: 3000
targetPort: 3000
nodePort: 30080
8. Step 5: Automating Deployment
Extend GitHub Actions to apply manifests after build:
deploy:
needs: build-and-push
runs-on: ubuntu-latest
steps:
- name: Checkout code
uses: actions/checkout@v3
- name: Set up Kubectl
uses: azure/setup-kubectl@v3
with:
version: 'v1.26.0'
- name: Apply manifests
run: |
kubectl apply -f k8s/deployment.yaml
kubectl apply -f k8s/service.yaml
9. Securing the Pipeline
- Use GitHub Secrets for credentials and tokens
- Never hardcode passwords in workflow files
- Use RBAC in Kubernetes to restrict access
- Limit image permissions in Docker Hub
10. Monitoring, Logging and Rollbacks
Monitoring:
- Prometheus + Grafana for metrics
- Kubernetes Dashboard for cluster health
- Sentry/New Relic for APM
Logging:
- ELK Stack (Elasticsearch + Logstash + Kibana)
- Loki + Grafana (lightweight alternative)
Rollbacks:
- Kubernetes supports easy rollbacks:
kubectl rollout undo deployment my-app
- Use Helm for versioned deployments
11. Tips for Production-Grade Pipelines
- Use Helm for templated deployments
- Integrate Slack or Microsoft Teams notifications
- Add unit/integration tests in your GitHub Actions
- Use canary deployments for safer rollouts
- Implement blue/green deployments
12. Final Thoughts
You’ve now created a production-grade DevOps CI/CD pipeline using some of the industry’s most trusted tools. This foundation enables your team to deliver software faster, more reliably, and with minimal human intervention.
Stay consistent with your DevOps practices, improve automation wherever possible, and keep your environments secure.
Happy deploying! 🚀